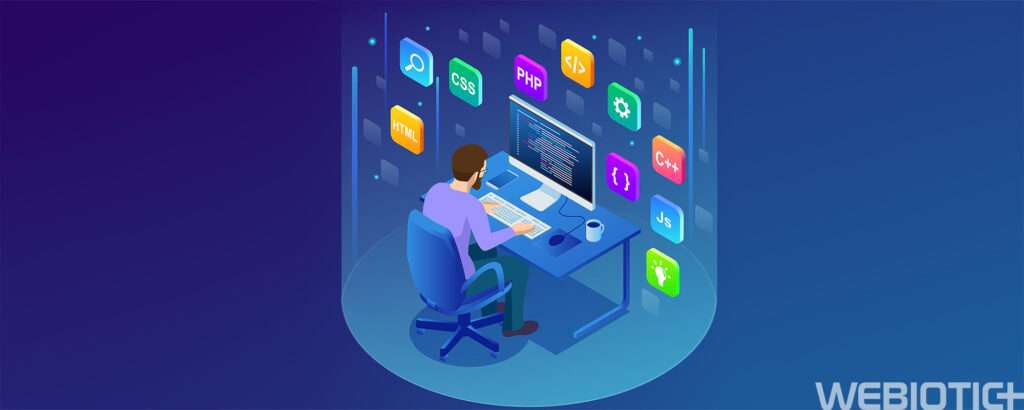
The Java programming language has been around since 1995 and has been the default language for Android app development since the platform was first introduced back in 2008.
Since then, Java has amassed enormous support and popularity in the dev community.
Even with the emergence of Kotlin as the new preferred language for Android mobile app development since 2019, Java is still widely used.
In this article, we’ll review why Java is still many developer’s go-to programming language and how it stacks up against other popular languages for Android, like Kotlin.
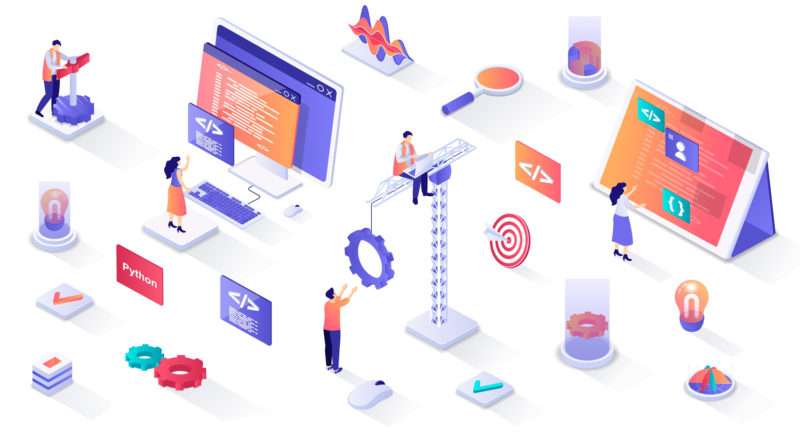
Table of Contents
- Java for Programmers
- Java Features
- Must-Haves for Java Mobile Development
- Android Studio
- NetBeans
- Gradle
- Eclipse
- Oracle JDeveloper
- Java vs Kotlin
- Kotlin
- Comparative Features
- Safety and Reliability
- Which is Better?
Java is a class-based, object-oriented programming language that was created to carry as few dependencies as possible so the compiled Java code could run on all Java-supported platforms without the need for recompilation.
In short, it’s always embraced the concept of “write once, run anywhere”.
Java was originally created by Sun Microsystems, which has since become Oracle Corporation. This language uses syntax that comes from other languages like C and C++.
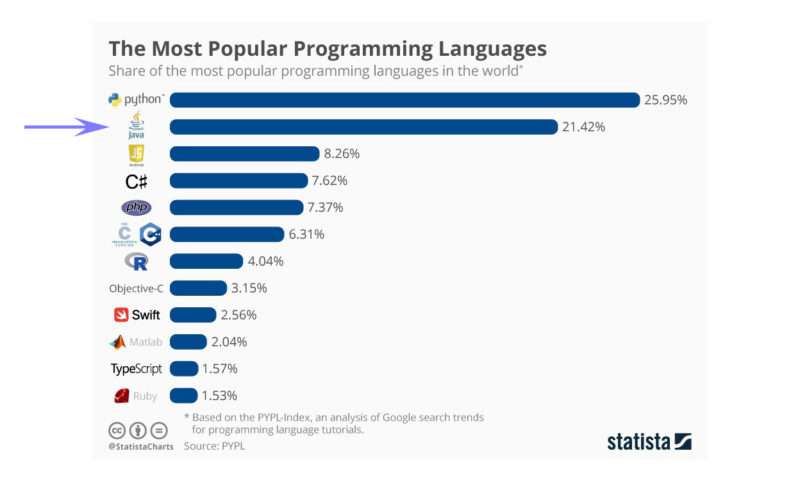
1.1 Java Features
Java has many features that make it a popular choice among both web and mobile app developers.
Let’s take a look at a few.
- Object-oriented: Just like with Python, C++, and Ruby, Java is an object-oriented programming language. Everything is an “object” that contains code and data.
- Platform independent: Since Java programs are first converted to bytecode by the Java compiler, the code can run on any machine that supports the Java runtime environment—making it platform-independent.
- Secure: Since Java is platform independent and has almost no interaction with the operating system, it makes the language much more secure than other programming languages.
- High-performing: While Java is an interpreted language, unlike C or C++ which are compiled, it nonetheless is high performing due to its own just-in-time compiler.
- Multi-threaded: With Java you can write applications that conduct multiple tasks in separate threads. For example, a Java app can serve users a login form while also running background processes.
- Open-source: Over the years, Java has amassed a great collection of open-source libraries that make java mobile apps much easier to develop.
- Community support: Since Java is an older programming language, it has a large community of developers that share valuable insights and knowledge when it comes to Java app development.
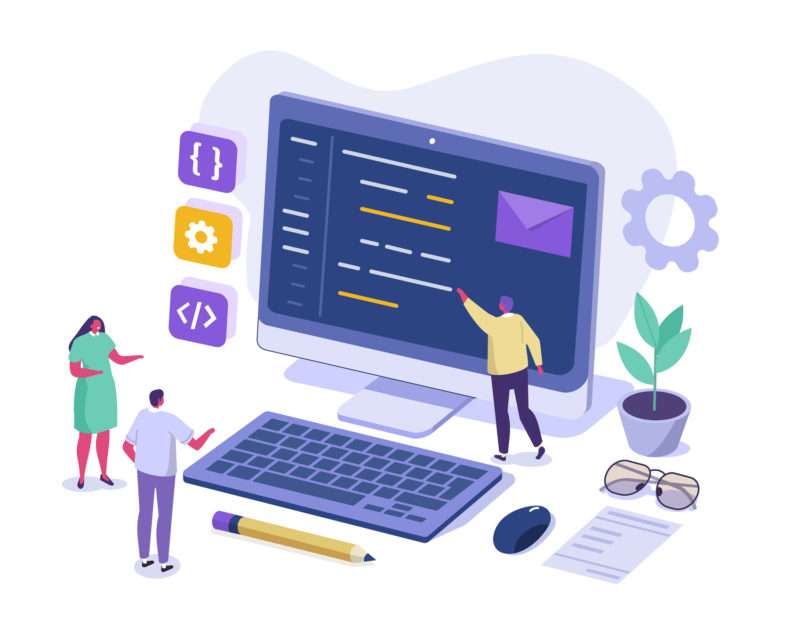
As discussed in the previous chapter, one of the greatest benefits to using Java is its wealth of open-source tools and libraries.
Here are some of the most widely used Java tools for building the best Java mobile apps.
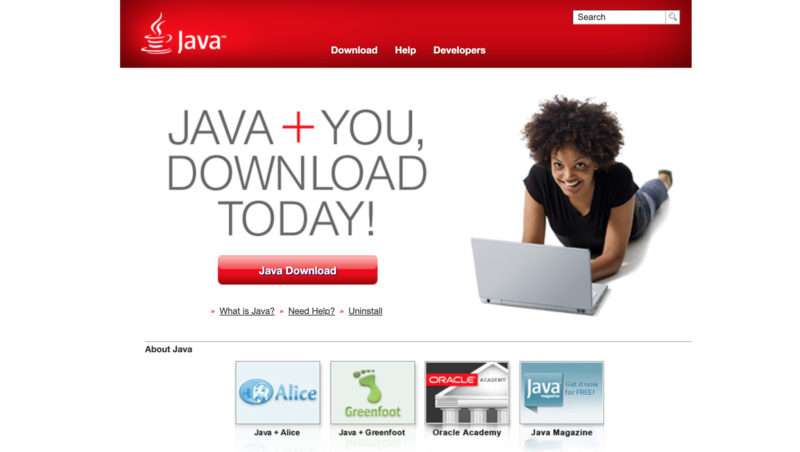
2.1 Android Studio
Android Studio is a must for developing Android mobile applications and is the official integrated development environment (IDE) for the Android platform.
Android Studio is based on IntelliJ IDEA, which is a Java-written IDE that’s designed to maximize productivity with its powerful code editor and developer tools.
Some additional features of Android Studio include a fast and feature-rich emulator, a flexible Gradle-based build system, a unified environment for developing apps for Android mobile devices, Lint tools, and so much more.
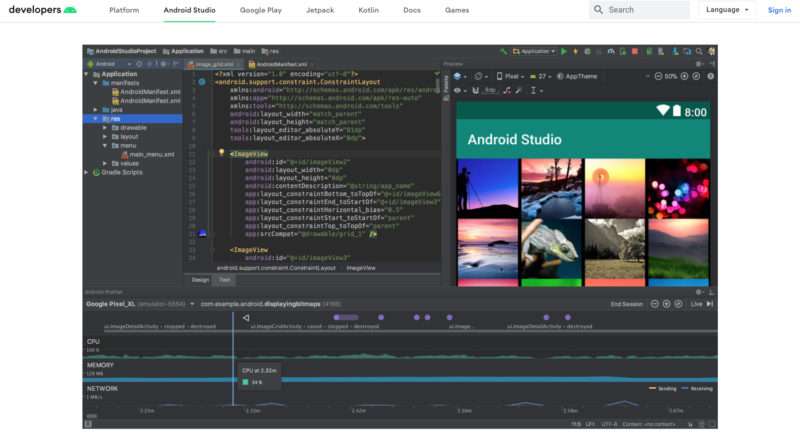
2.2 NetBeans
Just like Java itself, NetBeans is another product from Oracle Corporation. It’s an IDE designed for the Java language, and is completely free.
Developers can use NetBeans to create not only mobile applications but also desktop and web apps.
NetBeans runs on a modular architecture with a whole host of tools and features for the app development process, from idea inception to app store launch.
It comes with code analyzers, converters, visual debuggers, editors and more that help facilitate the app development cycle.
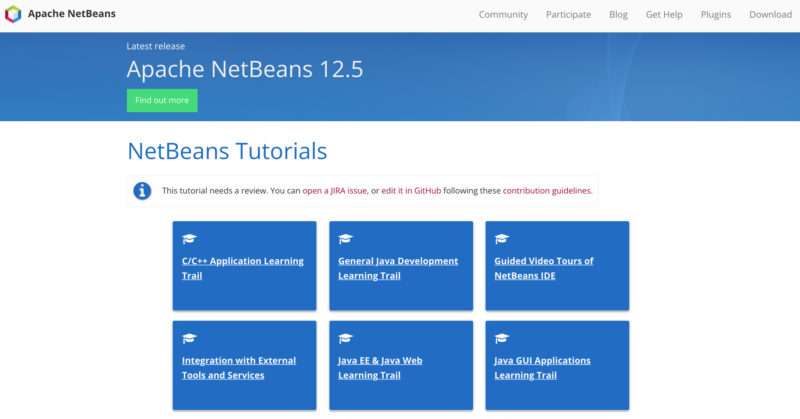
2.3 Gradle
Gradle is a flexible build automation tool that runs on the Java virtual machine (JVM) and requires a Java development kit.
Developers can use Java APIs in their build logic, like plugins and custom task types, and can use the tool on various platforms.
Since Gradle allows the use of a build cache to reuse task outputs from previous runs as well as other optimizations, it has high performance.
Additionally, most major IDEs like Android Studio and IntelliJ IDEA have the ability to import Gradle builds and interact with them.
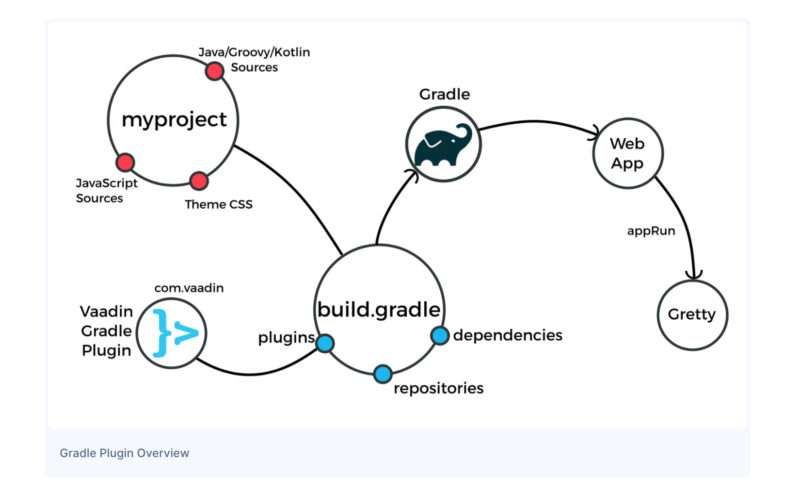
Photo Credit: vaadin.com
2.4 Eclipse
Eclipse is another popular IDE that provides assistance for code refactoring, syntax checking, and overall, code completion.
It also offers the Java Development Tools project (JDT) which contributes a set of plugins which add the capabilities of a full-fledged Java IDE to the Eclipse platform.
While Eclipse is famous for their Java IDE, they also offer a number of other helpful IDEs, like a C/C++ IDE, JavaScript/TypeScript IDE, PHP IDE and much more.
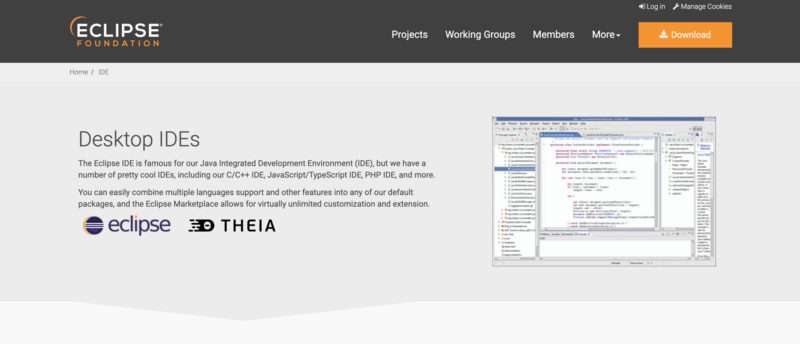
2.5 Oracle JDeveloper
Oracle JDeveloper is a completely free IDE that simplifies Java-based app development by addressing every step of the application cycle, from modeling and coding, to debugging, monitoring and deployment.
This IDE is optimized for the Oracle platform and assists with hand-coding, Java database connectivity (JBDC) compliance, cross-platform capabilities, testing, debugging and more.
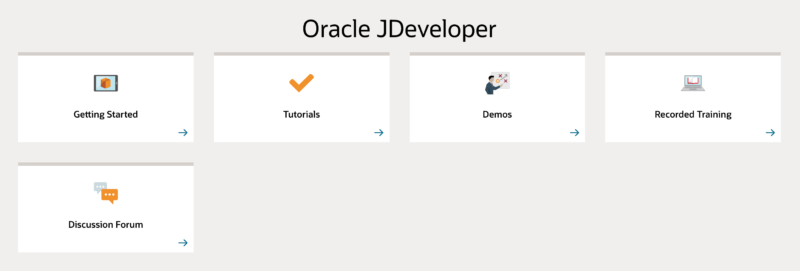
As already mentioned earlier, Kotlin has taken Java’s place as Google’s official language for Android app development, but that doesn’t mean developers have abandoned Java.
So which language is better and which should you use for developing mobile apps?
Let’s take a look at how Java and Kotlin stack up against each other and what each brings to the table
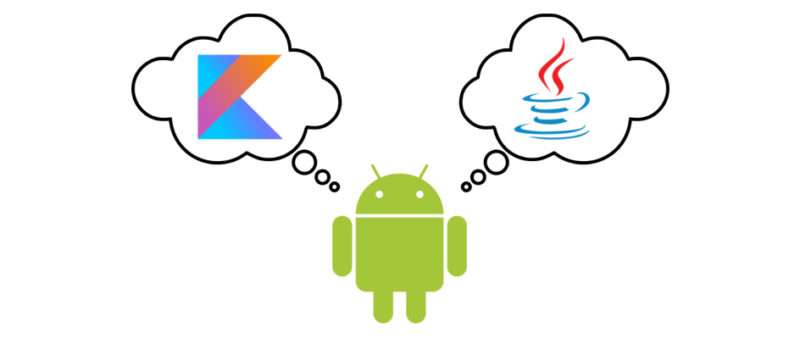
Photo Credit: codingflow.com
3.1 Kotlin
We’ve already reviewed what Java is and a few of its benefits, but what’s Kotlin?
Kotlin is a free, open-source programming language that runs on the JVM and is the official language of Android development.
Similar to Java, Kotlin can also be used in the same areas, including web, server, client, and Android applications development.
While it’s the youngest programming language out there, it’s proven to be a powerful one with robust features and clean code.
Now let’s dive into some differences between the two languages.
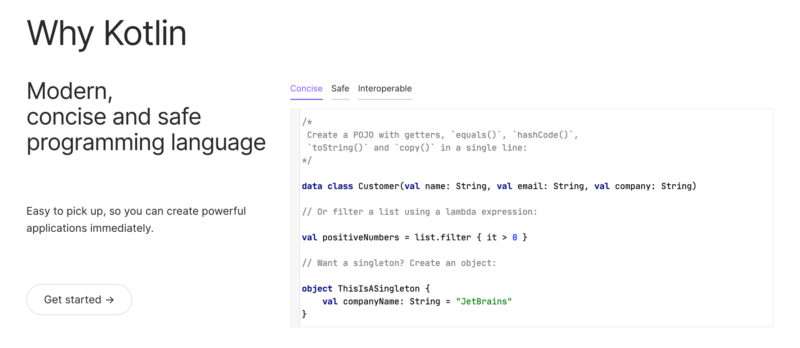
3.2 Comparative Features
Java and Kotlin, while interoperable, do have distinct features that cater to developers’ preferences and project needs.
Java, known for its robustness and widespread adoption, has a more verbose syntax which will often require more lines of code for tasks that Kotlin simplifies.
Kotlin, on the other hand, emphasizes conciseness and readability. Its modern syntax, influenced by other contemporary languages, often allows for more expressive coding in fewer lines.
Interoperability is another noteworthy feature; Kotlin seamlessly integrates with Java in the same project, providing flexibility for developers transitioning between the two.
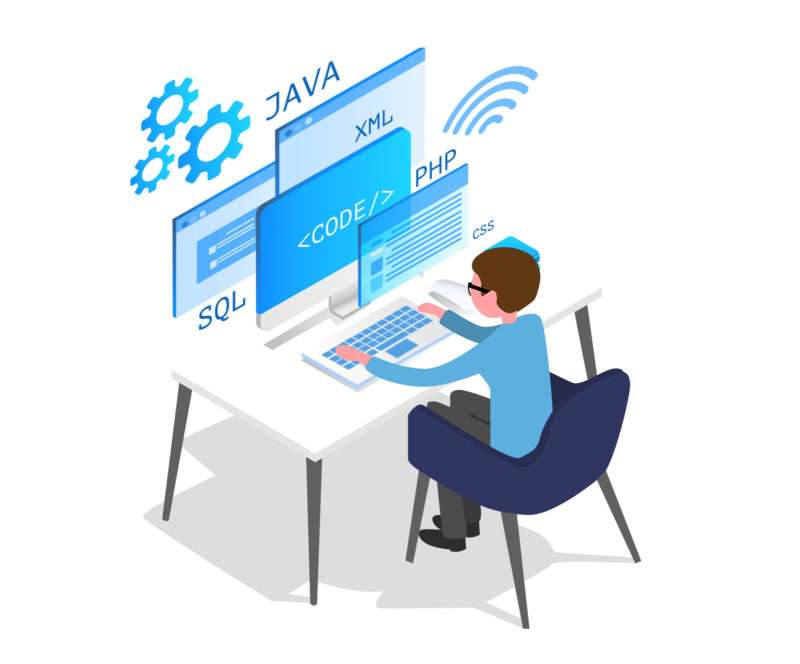
Performance-wise, both languages are comparable, especially in Android environments, though certain Kotlin features might offer slight runtime advantages.
Lastly, both have robust community support. While Java benefits from its longevity and vast developer base, Kotlin’s rapid adoption has fostered a vibrant, growing community dedicated to its advancement in the mobile app landscape.
3.3 Safety and Reliability
Java and Kotlin prioritize safety and reliability, albeit through differing approaches.
Java, with its established reputation, employs tried-and-tested mechanisms to tackle potential pitfalls, like the notorious null pointer exceptions.
But its lack of inherent null safety often necessitates developers to adopt rigorous practices to ensure null-related issues don’t come up.
Kotlin, recognizing the challenges posed by nullability, introduced built-in null safety features, making it harder to inadvertently introduce null reference bugs.
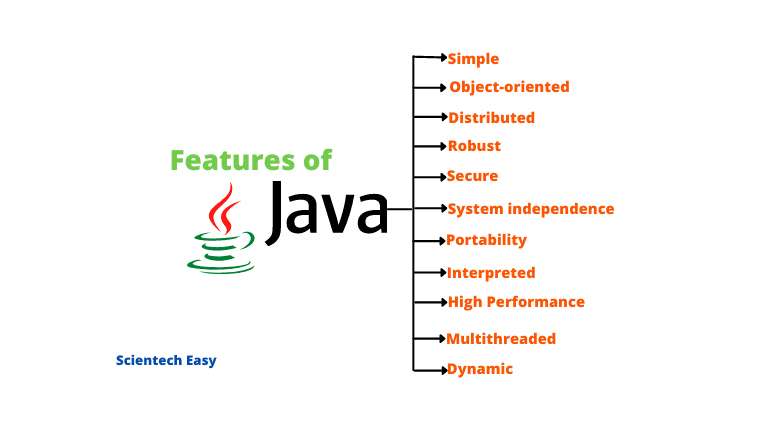
Kotlin’s type system inherently distinguishes nullable references from non-nullable ones, thereby reducing potential runtime crashes.
Exception handling also varies: Java’s checked exceptions demand explicit handling or propagation, while Kotlin lacks checked exceptions, relying on developers’ discretion for handling potential errors.
Both approaches have their advantages: Java’s method often ensures more predictability, while Kotlin’s offers flexibility.
3.4 Which is Better?
Choosing between Java and Kotlin isn’t quite a matter of absolute superiority; rather, it hinges on context and specific project requirements.
Java’s long-standing presence, extensive libraries, and broader ecosystem make it a reliable choice for large-scale, complex systems, especially where legacy integration is vital.
Kotlin, with its modern syntax, built-in null safety, and expressive code capabilities, is often favored for new Android projects, offering a streamlined development experience.
Additionally, Kotlin’s concise syntax can lead to fewer lines of code, potentially reducing the risk of bugs.
With that said, the “better” choice isn’t universal.
For teams well-versed in Java or maintaining existing Java applications, a switch to Kotlin might not always justify the learning curve or migration efforts.
Conversely, startups or new projects might benefit from Kotlin’s efficiencies and modern features.
Ultimately, the decision rests on aligning the language’s strengths with the project’s goals and the team’s expertise.
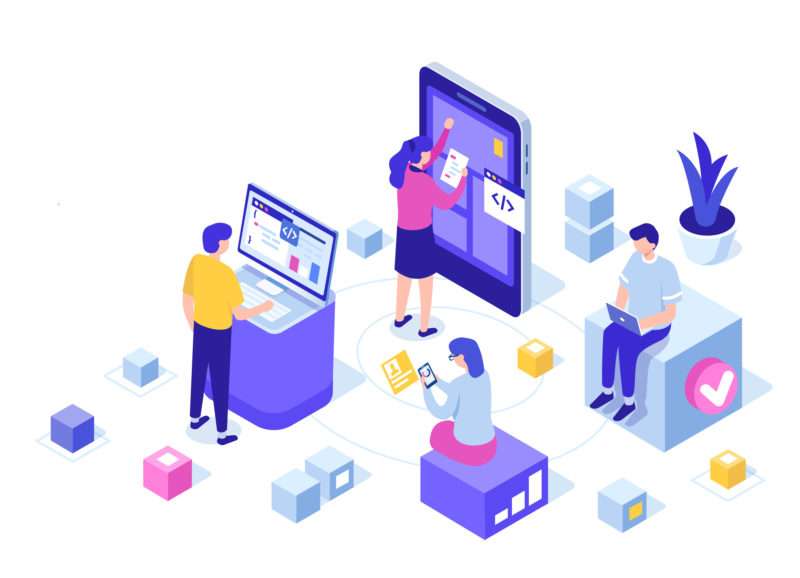
PRO TIP:
Kotlin has a steep learning curve and has a syntax that’s fairly different from Java, so going from Java to Kotlin would take time. If your dev team is proficient in Java, don’t expect a quick and easy transition to Kotlin.
Java is an incredibly powerful and well-established programming language with an enormous community and a wealth of libraries and tools that can help anyone build a mobile app.
It’s easy to learn, has cross-platform capabilities, and it’s secure, making it a language of choice for app development.
If you’re still unsure whether Java is right for your mobile app project, our Simple Starter package includes a full technical write up that includes details like the tech stack you’ll be using for your project, along with wireframes and market research.
What programming languages are you most loyal to and why?